Lesson 07: Inheritance
The idea of classes leads to the idea of inheritance. In our daily lives, we use the concept of classes being divided into subclasses. You know that the class of animals is divided into mammals, fishes, insects, birds, and so on. The class of vehicles is divided into cars, trucks, buses, and motorcycles.
The principle in this sort of division is that each subclass shares common characteristics with the class from which it's derived. Cars, trucks, buses, and motorcycles all have wheels and a motor and are used to transport people or goods; these are the defining characteristics of vehicles. In addition to the characteristics shared with other members of the class, each subclass also has its own particular characteristics: Buses, for instance, have seats for many people, whereas trucks have space for hauling heavy loads. The inheritance relationship is shown in Figure 1.
Figure 1: The Object Relationship of Vehicles
In a similar way, an OOP class can be used as the basis for one or more different subclasses. In C++, the original class is called the base class; other classes can be defined that share its characteristics, but add their own as well. These are called derived classes. The derived class inherits all the capabilities of the base class but can add embellishments and refinements of its own. The base class is unchanged by this process. The inheritance relationship is shown in Figure 2.
Figure 2: Inheritance
Inheritance Syntax
The simplest example of inheritance requires two classes: a base class and a derived class. The base class does not need any special syntax. The derived class, on the other hand, must indicate that it's derived from the base class. This is done by placing a colon (:) after the name of the derived class, followed by a keyword such as public and then the base class name.
The general syntax of a derived class is:
class className : accessSpecifier baseClassName
{
member_List;
};
Where accessSpecifier is public, protected, or private. When no accessSpecifier is specified, it is assumed to be a private inheritance. The colon (like the arrow in inheritance diagrams) means "is derived from."
Mode of Inheritance
When deriving a class from a base class, the base class may be inherited through public, protected, or private inheritance. The type of inheritance is specified by the access specifier as explained above.
We hardly use protected or private inheritance, but public inheritance is commonly used. While using a different type of inheritance, the following rules are applied:
Public Inheritance
When deriving a class from a public base class, public members of the base class become public members of the derived class, and protected members of the base class become protected members of the derived class. A base class's private members are never accessible directly from a derived class, but can be accessed through calls to the public and protected members of the base class.
Protected Inheritance
When deriving from a protected base class, public and protected members of the base class become protected members of the derived class.
Private Inheritance
When deriving from a private base class, public and protected members of the base class become private members of the derived class.
The private members in the base class cannot be directly accessed in the derived class, while protected members can be directly accessed. For example, Classes B, C, and D all contain the variables x, y, and z in the below example. It is just a question of access.
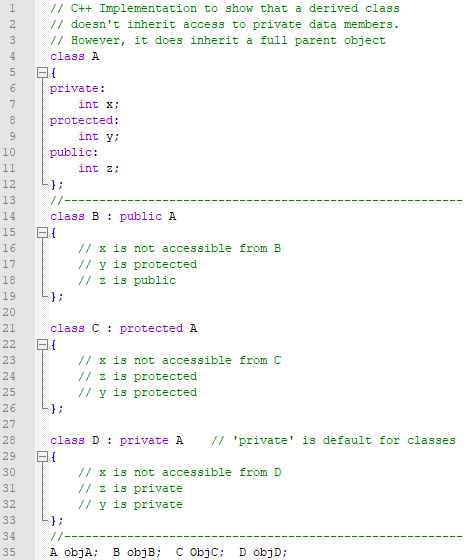
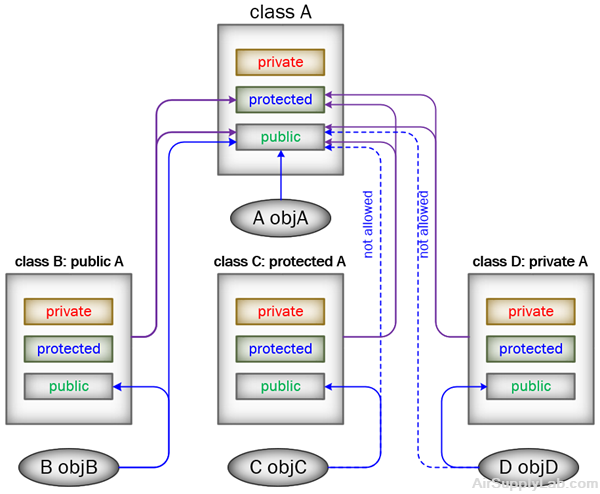
A derived class inherits all base class methods with the following exceptions:
- Constructors, destructors, and copy constructors of the base class.
- Overloaded operators of the base class.
- The friend functions of the base class.
Types of Inheritance
Single Inheritance
In single inheritance, a class is allowed to inherit from only one class. i.e. one sub class is inherited by one base class only.
class A
{ };
class B : public A
{ };

Multilevel Inheritance
Classes can be derived from classes that are themselves derived.
Here's a mini-program that shows the idea:
class A // base class A
{ };
class B : public A // B is derived from A
{ };
class C : public B // C is derived from B
{ };
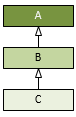
Here B is derived from A, and C is derived from B. The process can be extended to an arbitrary number of levels — D could be derived from C, and so on.
Multiple Inheritance
A class can be derived from more than one base class. This is called multiple inheritance. The syntax for multiple inheritance is similar to that for single inheritance.
The following code and diagram show how this looks when a class C is derived from base classes A and B.
class A // base class A
{ };
class B // base class B
{ };
class C : public A, public B // C is derived from A and B
{ };
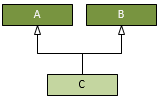
The base classes from which C is derived are listed following the colon (:) in C's specification; they are separated by commas (,).
Hierarchical Inheritance
In this type of inheritance, more than one sub class is inherited from a single base class. i.e. more than one derived class is created from a single base class.
class A // base class A
{ };
class B // base class B
{ };
class C // base class C
{ };
class D // base class D
{ };
class E : public A, public B // E is derived from A and B
{ };
class F : public C, public D // F is derived from C and D
{ };
class G : public E, public F // E is derived from E and F
{ };
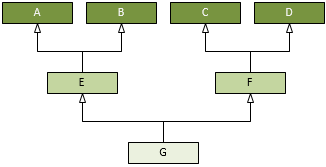
Hybrid (Virtual) Inheritance
Hybrid Inheritance is implemented by combining more than one type of inheritance. For example: Combining Hierarchical inheritance and Multiple Inheritance.
class A // base class A
{ };
class B // base class B
{ };
class C : public A, public B // C is derived from A and B
{ };
class D // base class D
{ };
class E : public C, public D // E is derived from C and D
{ };
class F : public D // F is derived from D
{ };
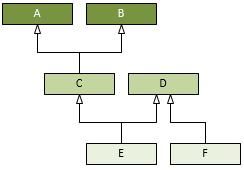
Multipath Inheritance
A derived class with two base classes and these two base classes have one common base class is called multipath inheritance. Ambiguity can arise in this type of inheritance.
class A // base class A
{ };
class B : public A // C is derived from A
{ };
class C : public A // C is derived from A
{ };
class D : public B, public C // D is derived from B and C
{ };
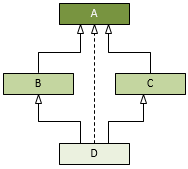
In the above example, both B and C inherit from A, they both have a single copy of A. However D inherits from both B and C, therefore D has two copies of A, one from B and another from C.
If object D needs to access the data member of A, the path from which A will be accessed must be specified: from B or C. Because the compiler cannot differentiate between two copies of A in D.
Example Code for Multipath Inheritance
Let us create a simple code for multipath inheritance followed by the above diagram.
The results of the above code are shown below:
a from class B : 10
a from class C : 20
b : 30
c : 40
d : 50
Mode of Inheritance
Mode of Inheritance